Today I got class... or used a class
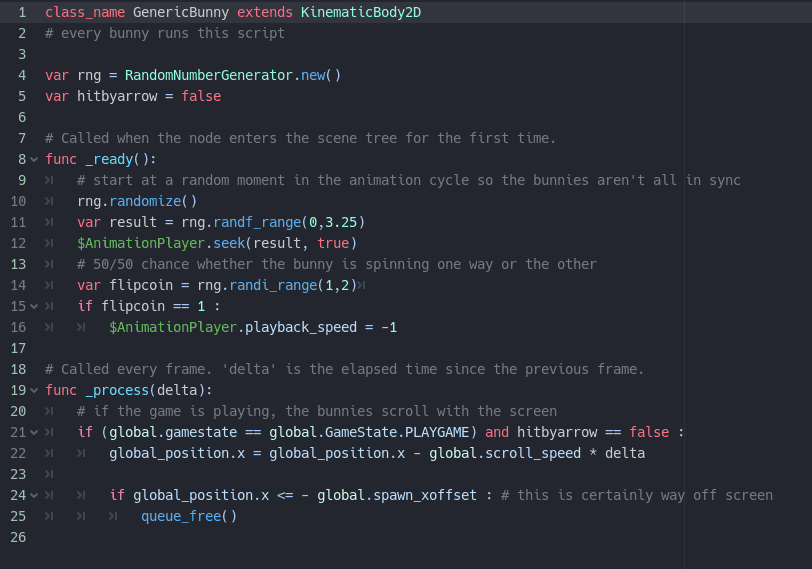
Today I figured out how to make a class for ALL the bunnies in GDScript so that the common code for all of them is in the same script file.
I still kinda think in TRS-80 Level II BASIC. Classes weren't a thing back then.
The programming I did for the NES was all in 6502 assembly.
So classes aren't really how I "think" about doing stuff, but this was a perfect place to use them. I wanted all of the bunnies to have the same code so that they scroll along with the background and queue_free() when they are off screen.
For more information, see this tutorial on classes:
Classes
Here's the script code without the fancy highlighting.
class_name GenericBunny extends KinematicBody2D
# every bunny runs this script
var rng = RandomNumberGenerator.new()
var hitbyarrow = false
# Called when the node enters the scene tree for the first time.
func _ready():
# start at a random moment in the animation cycle so the bunnies aren't all in sync
rng.randomize()
var result = rng.randf_range(0,3.25)
$AnimationPlayer.seek(result, true)
# 50/50 chance whether the bunny is spinning one way or the other
var flipcoin = rng.randi_range(1,2)
if flipcoin == 1 :
$AnimationPlayer.playback_speed = -1
# Called every frame. 'delta' is the elapsed time since the previous frame.
func _process(delta):
# if the game is playing, the bunnies scroll with the screen
if (global.gamestate == global.GameState.PLAYGAME) and hitbyarrow == false :
global_position.x = global_position.x - global.scroll_speed * delta
if global_position.x <= - global.spawn_xoffset : # this is certainly way off screen
queue_free()
With the above as the GenericBunny class, the script file for every different color bunny begins with:
extends GenericBunny
Do I need this? Maybe. If different color bunnies do something special, it's really easy for them to have that in their script file while making sure they all do the bunny things.